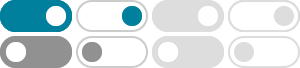
Introduction to Node.js
Node.js is an open-source and cross-platform JavaScript runtime environment. It is a popular tool for almost any kind of project! Node.js runs the V8 JavaScript engine, the core of Google Chrome, outside of the browser. This allows Node.js to be very performant. A Node.js app runs in a single process, without creating a new thread for every ...
Single executable applications | Node.js v23.10.0 Documentation
Users can create a single executable application from their bundled script with the node binary itself and any tool which can inject resources into the binary. Here are the steps for creating a single executable application using one such tool, postject: Create a JavaScript file: echo 'console.log(`Hello, ${process.argv[2]}!`);' > hello.js copy
Working with folders in Node.js
Use fs.access() (and its promise-based fsPromises.access() counterpart) to check if the folder exists and Node.js can access it with its permissions. Create a new folder. Use fs.mkdir() or fs.mkdirSync() or fsPromises.mkdir() to create a new folder.
C++ addons | Node.js v23.10.0 Documentation
When not using Node-API, implementing addons becomes more complex, requiring knowledge of multiple components and APIs: V8: the C++ library Node.js uses to provide the JavaScript implementation. It provides the mechanisms for creating objects, calling functions, etc.
Node-API | Node.js v23.11.0 Documentation
Binaries built with node-addon-api will depend on the symbols for the Node-API C-based functions exported by Node.js. node-addon-api is a more efficient way to write code that calls Node-API. Take, for example, the following node-addon-api code.
Writing files with Node.js
The easiest way to write to files in Node.js is to use the fs.writeFile() API. const fs = require ( ' node:fs ' ) ; const content = ' Some content! ' ; fs . writeFile ( ' /Users/joe/test.txt ' , content , err => { if ( err ) { console . error ( err ) ; } else { // file written successfully } } ) ;
Modules: CommonJS modules | Node.js v23.11.0 Documentation
CommonJS modules are the original way to package JavaScript code for Node.js. Node.js also supports the ECMAScript modules standard used by browsers and other JavaScript runtimes. In Node.js, each file is treated as a separate module.
Node.js — Introduction to TypeScript
For example, when you use Node.js with TypeScript, you'll need type definitions for Node.js APIs. This is available via @types/node. Install it using:
HTTP | Node.js v23.11.0 Documentation
This module, containing both a client and server, can be imported via require('node:http') (CommonJS) or import * as http from 'node:http' (ES module). The HTTP interfaces in Node.js are designed to support many features of the protocol which have been traditionally difficult to use.
Buffer | Node.js v23.11.0 Documentation
import { Buffer} from 'node:buffer'; // Create a single `Buffer` from a list of three `Buffer` instances. const buf1 = Buffer. alloc (10); const buf2 = Buffer. alloc (14); const buf3 = Buffer. alloc (18); …