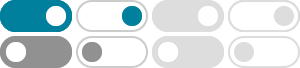
OpenTK - OpenTK
OpenTK The Open Toolkit is set of fast, portable, low-level C# bindings for OpenGL, OpenGL ES, OpenAL, and OpenCL. It runs on all major platforms and powers hundreds of apps, games, and scientific research programs.
LearnOpenTK - OpenTK
Welcome to LearnOpenTK, your home for getting started with OpenTK. We've ported the tutorials made by the great guys over at LearnOpenGL over to C# so that it's easier for you to see how OpenTK works.
Basics & Requirements - OpenTK
What is OpenTK? OpenTK is a library that provides high-speed access to native OpenGL, OpenCL, and OpenAL for .NET programs. OpenTK aims to make working with OpenGL, OpenCL, and OpenAL in .NET-supported languages like C# similar in both performance and feel to the equivalent C code, while still running in a managed (and safe!) environment.
Shaders - OpenTK
Shaders. As mentioned in the Hello Triangle Hello Triangle chapter, shaders are little programs that rest on the GPU. These programs are run for each specific section of the graphics pipeline. In a basic sense, shaders are nothing more than programs transforming inputs to outputs.
Creating a Window - OpenTK
Unlike OpenGL, OpenTK comes with its own windowing system. This tutorial will teach you how to use it. Go ahead and make a C# console project in your favourite IDE, and make a new file called Game.cs, and add the following using directives:
Transformations - OpenTK
We first define a vector named vec using OpenTK's built-in vector class. Next we define a Matrix4 and explicitly initialize it by calling the Matrix4.CreateTranslation function, which takes three floats and creates a translation matrix.
OpenGL - OpenTK
OpenGL. Note: No code will be written here. If you already know what OpenGL is and how it works, you can move straight to the next tutorial and get straight to programming.
Coordinate Systems - OpenTK
To create an orthographic projection matrix we make use of OpenTK's CreateOrthographicOffCenter method: Matrix4.CreateOrthographicOffCenter(0.0f, 800.0f, 0.0f, 600.0f, 0.1f, 100.0f); The first two parameters specify the left and right coordinate of the frustum and the third and fourth parameter specify the bottom and top part of the frustum.
Chapter 1 - OpenTK
This chapter contains the basics to get you up and running with OpenGL using OpenTK. This chapter contains the following parts: Introduction to OpenGL; Creating a window; Hello Triangle; Element Buffer Objects; Shaders; Textures; Multiple Textures; Transformations; Coordinate Systems; Camera
OpenTK API Reference
OpenTK API Reference. Welcome to the OpenTK API Reference. Here you can navigate through all of OpenTK's classes, namespaces, and other library members!